HOW CREATE XML DOCUMENTS WITH PHP?
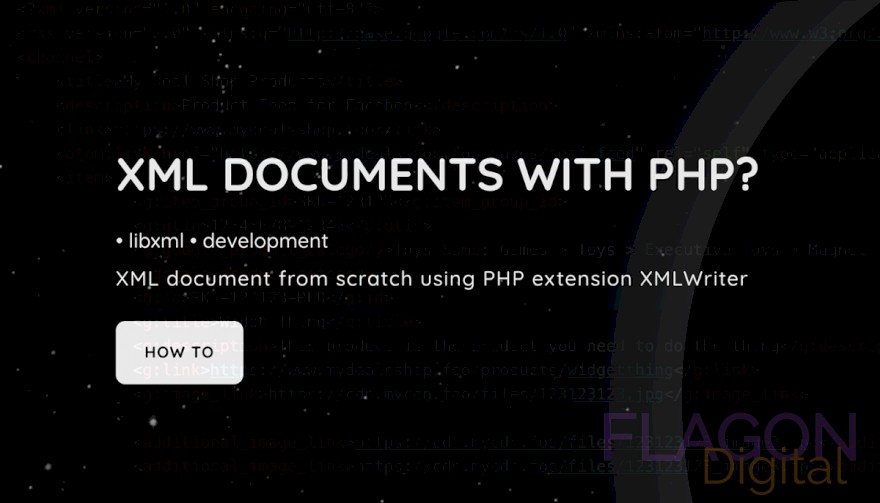
Many web applications need to work with XML files and feeds. Some examples include web services, remote catalog market products import, and scripts for data storage or data transfer.
XML stands for eXtensible Markup Language used to describe data. XML data is known as self-describing or self-defining, meaning that the structure of the data is embedded with the data, thus when the data arrives there is no need to pre-build the structure to store the data; it is dynamically understood within the XML. The XML format is widely used by marketplaces companies that want to import merchants information in a consistent way from online stores to large price comparison websites like pricecheck, bazaraki, google merchant (Google Shopping, formerly Google Product Search, Google Products and Froogle) and even facebook products catalog feed.
PHP have simple and clean xml manipulation functions like XMLWriter. XMLWriter is a wrapper for the libxml library.
Facebook products catalog XML feed example
Example Facebook accepted XML Feed (Atom rss) — for Commerce
<?xml version="1.0" encoding="utf-8"?>
<rss version="2.0" xmlns:g="http://base.google.com/ns/1.0" xmlns:atom="http://www.w3.org/2005/Atom">
<channel>
<title>My Deal Shop Products</title>
<description>Product Feed for Facebook</description>
<link>
https://www.mydealsshop.foo</link>
<atom:link href="https://www.mydealsshop.foo/pages/test-feed" rel="self" type="application/rss+xml" />
<item>
<g:item_group_id>SKU-123123</g:item_group_id>
<g:gtin>12345678912345</g:gtin>
<g:google_product_category>Toys & Games > Toys > Executive Toys > Magnet Toys</g:google_product_category>
<g:id>SKU-123123-RED</g:id>
<g:title>WidgetThing</g:title>
<g:description>This product is the product you need to do the thing</g:description>
<g:link>https://www.mydealsshop.foo/products/widgetthing</g:link>
<g:image_link>https://cdn.mycdn.foo/files/123123123.jpg</g:image_link>
<additional_image_link>https://cdn.mycdn.foo/files/123123123_image2.jpg</additional_image_link>
<additional_image_link>https://cdn.mycdn.foo/files/123123123_image3.jpg</additional_image_link>
<color>Red</color>
<additional_variant_attribute>
<label>Style</label>
<value>Cool</value>
</additional_variant_attribute>
<g:brand>AcmeCo</g:brand>
<g:condition>New</g:condition>
<g:availability>in stock</g:availability>
<g:price>19.99 USD</g:price>
<g:sale_price>9.99 USD</g:sale_price>
<g:offer_price>7.99 USD</g:offer_price>
<offer_price_effective_date>2018-07-25T01:35/2018-09-16T17:07</offer_price_effective_date>
</item>
</channel>
</rss>
Tell browser your document type with php
header('Content-Type: text/xml');
Start XML document in PHP
$xml = new XMLWriter();
$xml->openURI('php://output');
$xml->startDocument('1.0', 'UTF-8');
Create and open new XML element and close it
$xml->startElement('rss');
$xml->endElement();
Add attributes values to XML element
To add attributes to XML node modify code with writeAttribute
$xml->startElement('rss');
$xml->writeAttribute('version', '2.0')
$xml->writeAttribute('xmlns:g', 'http://base.google.com/ns/1.0')
$xml->endElement();
Add childrens XML elements
Every XML node element needs to be opened with the startElement method and closed with endElement.
$xml->startElement('rss');
$xml->writeAttribute('version', '2.0')
$xml->writeAttribute('xmlns:g', 'http://base.google.com/ns/1.0')
$xml->startElement('channel');
$xml->startElement('title');
$xml->endElement();
$xml->endElement();
$xml->endElement();
Add text inside XML elements
Depending on your application XML node have inner text
$xml->startElement('rss');
$xml->writeAttribute('version', '2.0')
$xml->writeAttribute('xmlns:g', 'http://base.google.com/ns/1.0')
$xml->startElement('channel');
$xml->startElement('title');
$xml->text('My Deal Shop Products');
$xml->endElement();
$xml->endElement();
$xml->endElement();
XMLReader
PHP XMLWriter class can be used for writing documents only. If you need to read an existing XML document, you have to use the XMLReader class
XMLWriter class vs PHP echo
How to echo an PHP xml without XMLWriter functions? Output as any large strings with dot sign to divide lines and "\n"
or PHP_EOL
next new line character
Header('Content-Type: text/xml');
$XML_line = '<?xml version="1.0" encoding="UTF-8"?>'."\n";
$XML_line .= '<rss version="2.0" xmlns:g="http://base.google.com/ns/1.0" xmlns:atom="http://www.w3.org/2005/Atom">'."\n";
$XML_line .= '<channel>'."\n";
$XML_line .= "\t".'</channel>'."\n";